Creating an entity
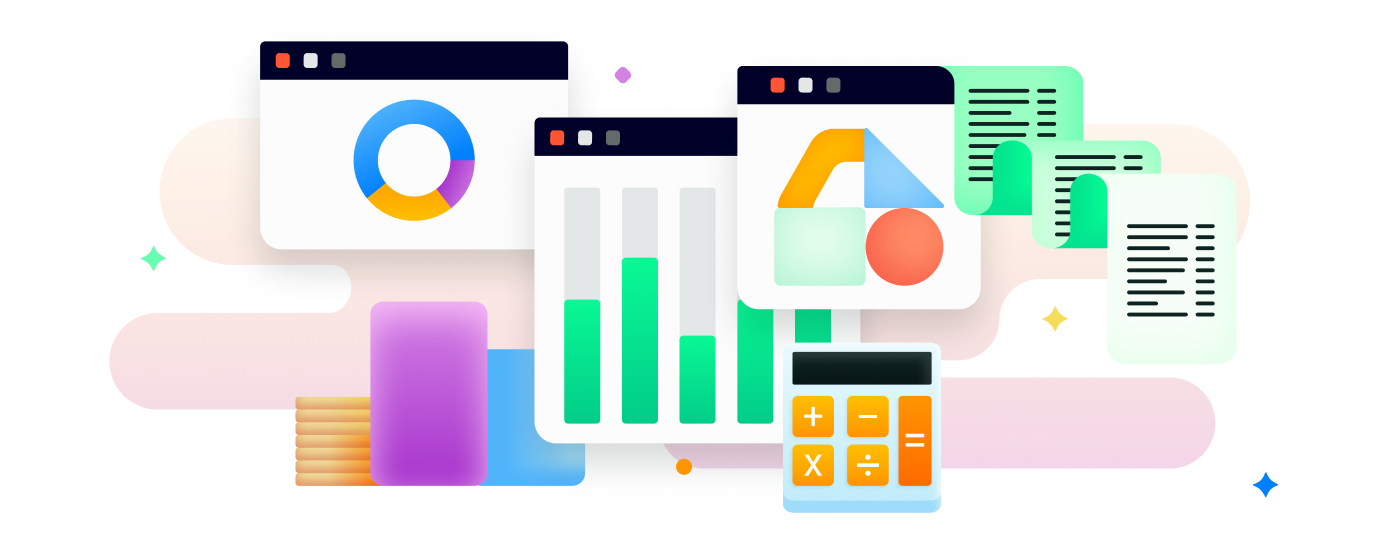
An Entity maps to a user's entire relationship with a specific bank. An example Entity object will look something like the following:
{
"id": "f6dcc0ae-20c6-318c-bdba-49fb2ced41ce",
"customer_id": "6bc2336a-6d74-4e59-a492-65313423a8f8",
"bank_identifier": "LEA1_RETAIL_SAU",
"account_type": "BUSINESS" | "PERSONAL",
"permissions": {
"identity": true,
"accounts": true,
"balance": true,
"transactions": true,
"identities": true,
"scheduled_payments": true,
"standing_orders": true,
"direct_debits": true,
"beneficiaries": true,
},
"consents": [
{
"id": "5f5ece67-e029-49d5-8125-05863d072597",
"bank_consent_id": "abcd-1000",
"consent_status": "ACTIVE",
"permissions": {
"identity": true,
"accounts": true,
"balance": true,
"transactions": true,
"identities": true,
"scheduled_payments": true,
"standing_orders": true,
"direct_debits": true,
"beneficiaries": true
},
"creation_date_time": "2023-03-07T09:33:30.477Z",
"status_update_date_time": "2023-03-07T09:33:50.907876Z",
"expiration_date_time": "2023-09-07T09:33:30.403933Z",
"transaction_from_date_time": "2022-09-07T09:33:30.403Z",
"transaction_to_date_time": "2023-09-07T09:33:30.403Z",
"accounts": [
{
"scheme_name": "IBAN",
"identification": "SA4915000900379446000009",
"name": "ABDULRAHMAN ABDULLAH A ALRAHMA",
"secondary_identification": null
}
]
}
]
}
Entities are created when your customer completes the bank account connection flow through our LinkSDK.
Consent explanation
Before a customer is prompted to connect their bank account, you will need to explain to them what they are consenting to. Please refer to the Consent explanation guide on how to do this.
Connecting a bank account
In order to allow your customer to connect their account, you will need to call theLean.connect()
method in the LinkSDK in your front-end:
<button onClick={connectAccount([customer_id])}>Connect Account</button>
<script>
function connectAccount = (customer_id) => {
Lean.connect({
app_token: "YOUR_APP_TOKEN",
customer_id: customer_id,
permissions: [
"identity","accounts","balance","transactions", "identities",
"scheduled_payments", "standing_orders", "direct_debits", "beneficiaries"
],
sandbox: true,
fail_redirect_url: "",
success_redirect_url: "",
access_from: "2023-03-07T09:33:30.477Z", //optional
access_to: "2023-06-07T09:33:30.477Z", //optional
account_type: "BUSINESS" | "PERSONAL"
})
}
</script>
In the call, you will need to provide, at a minimum, the permissions for the endpoints you want to make calls for, your app_token
and a customer_id
. We will explain in the following sections the optional paramaters access_to
, access_from
, fail_redirect_url
and success_redirect_url
.
Please see LinkSDK section for more details on integrating the Link SDK into your application and additional functionality.
Define the timeframe you are requesting data for
In addition, you can set access_from
, which determines how long back you will be allowed to retrieve the data from the bank account, and access_to
, which determines when the consent will expire. If you do not set access_from
or access_to
you will be able to retrieve all the data the bank can provide for an indefinite amount of time. Both access_from
and access_to
are datetimes that can be set with timezone but if you do not provide a timezone, the datetimes will be assumed to be in local KSA time.
Possible values for access_to
The following are the possible values for access_to
:
- If you want to request consent to access the data only once (single use consent), you will need to set
access_to
to a maximum of 24 hours from the time you are requesting the consent - If you want to request a consent to access the data multiple times but not indefinitely (long lived consent), you will need to set
access_to
to be more than 24 hours and up to 1 year from the time you are requesting the consent - If you want to request a consent to access the data indefinitely (open-ended consent), you will not set
access_to
The following are values that are NOT allowed for access_to
:
- Any value that is before the time you are requesting the consent as this would mean that the consent generated would be already expired when created
- Any value that is after 1 year of the time you are requesting the consent as this is not allowed by SAMA's Open Banking Framework
Note that irrespective of the value of access_to
you set, your customer will be able to revoke the consent whenever they choose to.
Possible values for access_from
The following are the possible values for access_from
:
- If you want to access all the historical transactions for the bank account, you will not set
access_from
- If you want to request the historical transactions data up to a certain point back in time, you will set
access_from
to be that point in the past - If you want to access any data for the bank account but not transactions, you will not set
access_from
as this setting only applies to transactions data
The following are values that are NOT allowed for access_from
:
- Any value that is after the value set for
access_to
as this would be incoherent
Redirection of end users to and from their bank
Once the customer has selected a bank and gone through the Link SDK they will be redirected to their bank's website. Here they can confirm the authenticity of the website by validating the SSL encryption.
You will also need to set fail_redirect_url
and success_redirect_url
in .connect()
to define where you would like your customer to be redirected once the consent has failed or has been successfully set up. These can be deep links so the end user returns right back to your iOS or Android app. We will send you more details about any error as query parameters in fail_redirect_url
.
Your customer will then go through the LinkSDK where they can select their bank account and will then be redirected to the bank's app or website and will then be able to select which accounts to share.
After the customer completes the LinkSDK
When a customer completes the LinkSDK - please note, no details are shared directly from the SDK to your front-end. A Success, Error or Cancelled message can be returned from the SDK via a callback (depending on the platform you're integrating with, this is handled differently) in the following format:
{
status: "SUCCESS",
method: "LINK",
message: "Customer created entity successfully"
}
The details of your newly created entity will instead be sent via a webhook to your backend services. Your front-end should therefore handle the success by making a call to your backend to refresh the data.
When a customer successfully links their bank with your application a webhook is sent back to your application:
{
"type": "entity.created",
"message": "An entity object has been created.",
"payload": {
"id": "f08fb010-878f-407a-9ac2-a7840fb56185",
"customer_id": "6bc2336a-6d74-4e59-a492-65313423a8f",
"permissions": [
"identity","accounts","balance","transactions", "identities",
"scheduled_payments", "standing_orders", "direct_debits", "beneficiaries"
],
"bank_details": {
"name": "Lean SAMA Open Banking MockBank",
"identifier": "LEA1_SAMAOB_SAU",
"logo": "https://cdn.leantech.me/img/banks/white-lean.png",
"main_color": "#1beb75",
"background_color": "#001E26"
}
},
"timestamp": "2020-10-10T17:19:00.059933Z"
}
The new Entity details can be found under payload in the received webhook. It is up to you which details from this webhook you decide to save to your database, but at the minimum we suggest you save the entity_id
for future use.
It's also worth noting that a user can have multiple connected entities - one for each bank they have an account for.
Updated 2 months ago